Unity Observer
Observer panel that invokes methods, observes and changes values within the editor, making tests easier. Especially useful for testing variables, debugging values, and invoking methods to streamline development.
Unity Observer is an editor window that allows you to invoke methods, observe values, and change them within the Unity editor's play mode. This tool is designed to facilitate testing changes, observing values, and fast-invoking functions, significantly improving overall testing efficiency.
I developed Unity Observer as a side project and successfully battle-tested it in Stygian: Reign of the Old Ones.
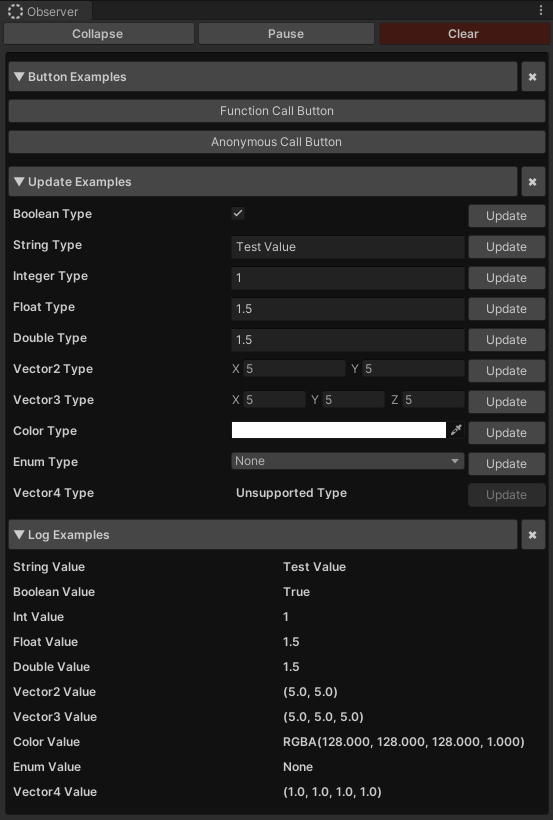
Log
The Log function allows you to log values using key-value pairs, which are displayed in real-time.
csharp
Observer.Log("Log Examples", "String Value", "Test String");
Observer.Log("Log Examples", "Boolean Value", true);
Observer.Log("Log Examples", "Int Value", 150);
Observer.Log("Log Examples", "Float Value", 1.50f);
Observer.Log("Log Examples", "Double Value", 1.50);
Observer.Log("Log Examples", "Vector2 Value", new Vector2(2,2));
Observer.Log("Log Examples", "Vector3 Value", new Vector3(2, 2, 2));
Observer.Log("Log Examples", "Color Value", new Color(128, 128, 128));
Observer.Log("Log Examples", "Enum Value", MyEnum.MyValue);
Value
The Value function allows you to update a value using callbacks. It supports multiple types for display.
csharp
// Boolean Example
Observer.Value("Update Examples", "Boolean Type", m_BoolTest, (object val) =>
{
m_BoolTest = (bool)val;
});
// String Example
Observer.Value("Update Examples", "String Type", m_StringTest, (object val) =>
{
m_StringTest = (string)val;
});
// Integer Example
Observer.Value("Update Examples", "Integer Type", m_IntTest, (object val) =>
{
m_IntTest = (int)val;
});
// Float Example
Observer.Value("Update Examples", "Float Type", m_FloatTest, (object val) =>
{
m_FloatTest = (float)val;
});
// Double Example
Observer.Value("Update Examples", "Double Type", m_DoubleTest, (object val) =>
{
m_DoubleTest = (double)val;
});
// Vector2 Example
Observer.Value("Update Examples", "Vector2 Type", m_Vec2Test, (object val) =>
{
m_Vec2Test = (Vector2)val;
});
// Vector3 Example
Observer.Value("Update Examples", "Vector3 Type", m_Vec3Test, (object val) =>
{
m_Vec3Test = (Vector3)val;
});
// Color Example
Observer.Value("Update Examples", "Color Type", m_ColorTest, (object val) =>
{
m_ColorTest = (Color)val;
});
// Enum Example
Observer.Value("Update Examples", "Enum Type", m_EnumTest, (object val) =>
{
m_EnumTest = (ObserverEntryType)val;
});
Button
The Button function allows you to register a button and invoke a given function.
csharp
// Calls a function
Observer.Button("Button Examples", "Function Call Button", TestFunction);
// Anonymous function
Observer.Button("Button Examples", "Anonymous Call Button", () =>
{
Debug.Log("Hello, World!");
});